C++带有线程操作,异步操作,就是没有线程池。一般而言,当你的函数需要在多线程中运行,但是你又不能每来一个函数就开启一个线程,所以你就需要根据资源情况固定几个线程来执行,但会出现有的线程还没有执行完,有的又在空闲,如何分配任务呢?这时你就需要封装一个线程池来完成这些操作,有了线程池这层封装,你就只需要告诉它开启几个线程,然后直接往里塞任务就行,并通过一定的机制获取执行结果。
下边是一个简单的C++11实现的线程池,原理是管理一个任务队列和一个工作线程队列,工作线程不断的从任务队列取任务,然后执行;如果没有任务就挂起休眠等待新任务的到来。添加新任务的时候先添加到任务队列,然后通知任意一个线程notify_one『别睡了,起来嗨!』。
源代码来自https://github.com/progschj/ThreadPool,有3.9K的start,废话不多说,直接分析代码。
线程池:
/*
* threadpool.h
* 线程池
*/
#pragma once
#include <vector>
#include <queue>
#include <memory>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <future>
#include <functional>
#include <stdexcept>
namespace mars {
namespace util {
class ThreadPool {
public:
// 构造函数,传入线程数
ThreadPool(size_t);
// 入队任务(传入函数和函数的参数)
template<class F, class... Args>
auto enqueue(F&& f, Args&&... args) //任务管道函数
-> std::future<typename std::result_of<F(Args...)>::type>; //利用尾置限定符 std::future用来获取异步任务的结果
// 析构
~ThreadPool();
private:
// 工作线程组 need to keep track of threads so we can join them
std::vector< std::thread > workers;
// 任务队列 the task queue
std::queue< std::function<void()> > tasks;
// 异步 synchronization
std::mutex queue_mutex; // 队列互斥锁
std::condition_variable condition; // 条件变量
bool stop; // 停止标志
};
// 构造函数仅启动一些工作线程 the constructor just launches some amount of workers
inline ThreadPool::ThreadPool(size_t threads)
: stop(false)
{
for(size_t i = 0; i< threads; ++i)
// 添加线程到工作线程组
workers.emplace_back( // 与push_back类似,但性能更好(与此类似的还有emplace/emlace_front)
[this]
{
for(;;) // 死循环,线程内不断的从任务队列取任务执行(这样写比while(true)效率更高?)
{
std::function<void()> task; //线程中的函数对象
// 通过lock互斥获取一个队列任务和任务的执行函数(大括号作用:控制lock临时变量的生命周期,执行完毕或return后自动释放锁)
{
// 拿锁(独占所有权式)
std::unique_lock<std::mutex> lock(this->queue_mutex);
// 等待条件成立(当线程池被关闭或有可消费任务时跳过wait继续;否则condition.wait将会unlock释放锁,其他线程可以继续拿锁,但此线程会阻塞此处并休眠,直到被notify_*唤醒,被唤醒时wait会再次lock并判断条件是否成立,如成立则跳过wait,否则unlock并休眠继续等待下次唤醒)
this->condition.wait(lock, [this]{ return this->stop || !this->tasks.empty(); });
// 如果线程池停止且任务队列为空,说明需要关闭线程池结束返回
if(this->stop && this->tasks.empty())
return;
// 取得任务队首任务(注意此处的std::move)
task = std::move(this->tasks.front());
// 从队列移除
this->tasks.pop();
}
// 调用函数执行任务(执行任务时lock已释放,不影响其他线程取任务)
task();
}
}
);
}
// 添加一个新的工作任务到线程池 add new work item to the pool
template<class F, class... Args>
auto ThreadPool::enqueue(F&& f, Args&&... args)
-> std::future<typename std::result_of<F(Args...)>::type>
{
using return_type = typename std::result_of<F(Args...)>::type;
// 将任务函数和其参数绑定,构建一个packaged_task(packaged_task是对任务的一个抽象,咱们能够给其传递一个函数来完成其构造。以后将任务投递给任何线程去完成,经过packaged_task.get_future()方法获取的future来获取任务完成后的产出值)
auto task = std::make_shared< std::packaged_task<return_type()> >( //指向F函数的智能指针
std::bind(std::forward<F>(f), std::forward<Args>(args)...) //传递函数进行构造
);
// 获取任务的future
std::future<return_type> res = task->get_future();
{
// 独占拿锁
std::unique_lock<std::mutex> lock(queue_mutex);
// 不允许入队到已经停止的线程池 don't allow enqueueing after stopping the pool
if(stop)
throw std::runtime_error("enqueue on stopped ThreadPool");
// 将任务添加到任务队列
tasks.emplace([task](){ (*task)(); });
}
// 发送通知,唤醒一个wait状态的工作线程重新抢锁并重新判断wait条件
condition.notify_one();
return res;
}
// 析构线程池 the destructor joins all threads
inline ThreadPool::~ThreadPool()
{
{
// 拿锁
std::unique_lock<std::mutex> lock(queue_mutex);
// 停止标志置true
stop = true;
}
// 通知所有工作线程重新抢锁并重新判断wait条件,唤醒后因为stop为true了,所以都会结束
condition.notify_all();
// 等待所有工作线程结束
for(std::thread &worker: workers)
worker.join(); //由于线程都开始竞争了,因此必定会执行完,join可等待线程执行完
}
}
}
线程池测试:
/*
* test threadpool
*
* 编译:
* g++ test_threadpool.cc -std=c++11 -pthread -o build/test_threadpool
*/
#include <iostream>
#include <vector>
#include <chrono>
#include "mars/util/threadpool.h"
int main(){
LOG(INFO) << "ThreadPool:";
mars::util::ThreadPool pool(4); //线程池
std::vector< std::future<int> > results; //线程执行结果
// 批量执行线程任务
for(int i = 0; i < 8; ++i) {
results.emplace_back( // 保存线程执行结果到results
pool.enqueue([i] { // 添加一个新的工作任务到线程池
std::cout << "hello " << i << std::endl;
//std::this_thread::sleep_for(std::chrono::seconds(1));
//std::cout << "world " << i << std::endl;
return i*i;
})
);
}
// 打印线程执行结果
for(auto && result: results)
std::cout << "result:" << result.get() << std::endl;
std::cout << std::endl;
return 0;
}
//执行结果
I1230 22:43:38.986207 234823104 main.cc:511] ThreadPool:
hello hello hello 21
hello 0result:
3
hello hello 5
hello 6
hello 7
4
0
result:1
result:4
result:9
result:16
result:25
result:36
result:49
参考:
C++11 并发指南四(<future> 详解二 std::packaged_task 介绍)
C++11 并发指南四(<future> 详解三 std::future & std::shared_future)
C++11 并发指南五(std::condition_variable 详解)
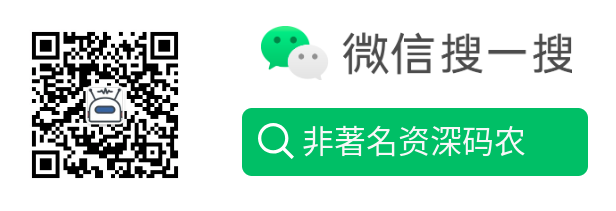